Understanding the Bottlenecks: Python's GIL and the Limitations of Current ML Infrastructure
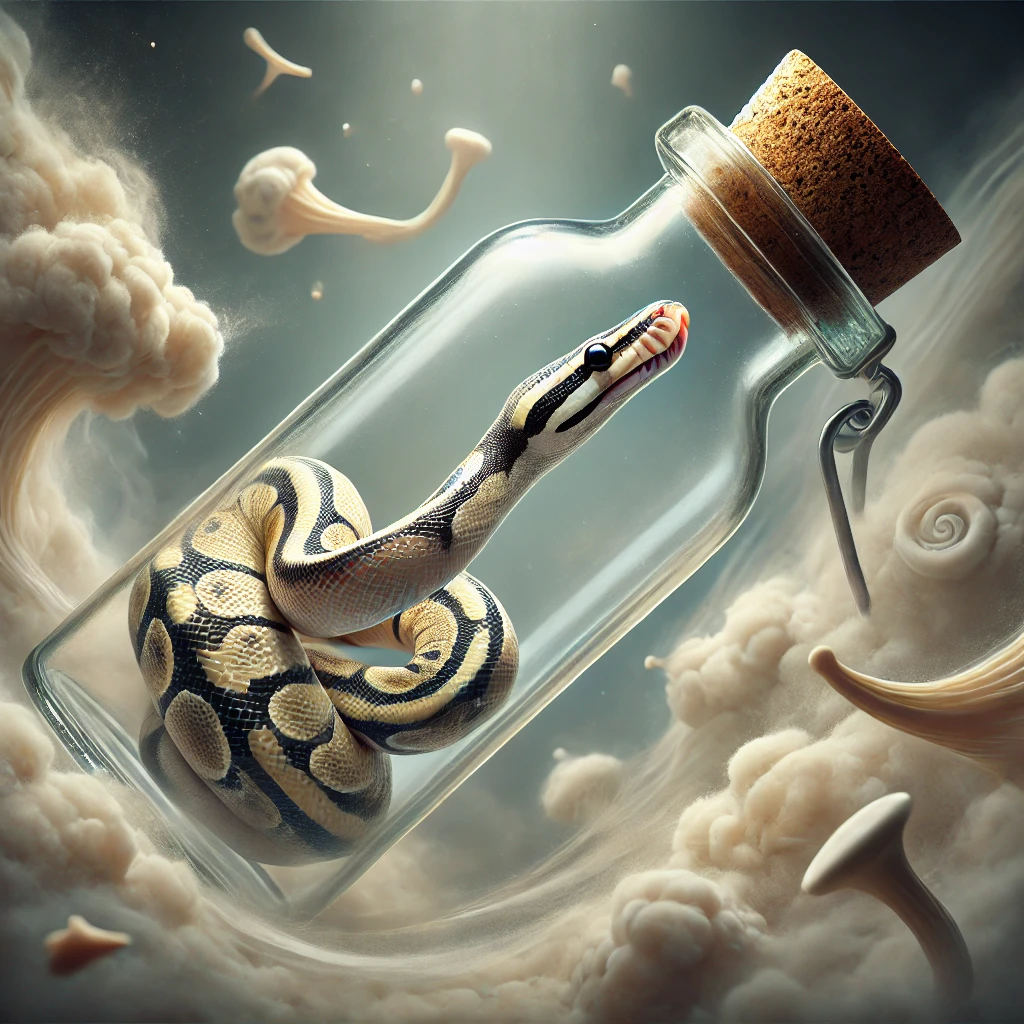
The Hidden Roadblock in Python's Core
At the heart of Python's architecture lies a mechanism that most developers rarely think about until it becomes a performance bottleneck: the Global Interpreter Lock (GIL). This seemingly innocuous component has profound implications for machine learning workloads and high-performance computing. The Python GIL is a mutex/lock that allows only one thread to hold the control of the Python interpreter and thereby it's orchestration and execution. This means that only one thread can be in a state of execution at any point in time. While this design choice made perfect sense in Python's early days, it has become increasingly problematic as hardware capabilities have evolved toward multi-core architectures.
Understanding the GIL: A Technical Perspective
To appreciate why the GIL exists, we must first understand Python's memory management system. Python uses reference counting for memory management, meaning objects created in Python have a reference count variable that tracks the number of references pointing to the object. When this count reaches zero, the memory occupied by the object is released. This approach simplifies memory management, but introduces a critical vulnerability: race conditions in a multi-threaded environment.
The problem emerges when multiple threads attempt to modify the same reference count simultaneously. Without protection, this could lead to memory leaks or, worse, premature object deletion while references still exist. The GIL was implemented as a straightforward solution to this problem, ensuring thread safety by allowing only one thread to execute Python bytecode at a time, effectively serializing Python operations regardless of the number of available CPU cores.
The Real-World Impact on Machine Learning Workloads
The GIL's constraints become particularly evident in CPU-bound machine learning applications. In machine learning contexts, "real time systems, where large volumes of data need to be processed simultaneously, may face limitations due to the GIL... since these systems usually demand high-performance processing and often involve multi-threading." This creates a significant bottleneck exactly where performance matters most: computationally intensive tasks like model training, hyperparameter optimization, and feature engineering. There is a strong and continually growing argument to be made that we shouldn't have to make such a significant trade off in the modern era, and worse - given the volume of data needing processing and analyzed, we can't readily accept that exchange rate off going forward especially for production and critical systems that necessitate at or near real time response.
In practical terms, this means that even on a 64-core server, a Python process will primarily utilize just one core for executing Python code, leaving substantial computing power idle. This limitation is especially frustrating given the inherently parallelizable nature of many machine learning algorithms. Tasks like matrix operations, gradient calculations, and batch processing would ideally benefit from parallel execution, yet Python's GIL prevents efficient utilization of multiple cores.
Even C/C++ Extensions Feel the GIL's Grip
A common misconception is that using C/C++ extensions in Python completely bypasses the GIL's limitations. While these extensions can release the GIL during execution, the reality is more nuanced. To Wit, locking the entire interpreter makes it easier for the interpreter to be multi-threaded, at the expense of much of the parallelism afforded by multi-processor machines. This means that even when using optimized libraries like NumPy, TensorFlow, or PyTorch (which are largely implemented in C/C++), the Python code coordinating these libraries still runs under the GIL's constraints. This has been a commonly known concern and accepted reality within the Python community with some amazing conversations and insights about it including David Beazly and Jesse Noller (Note: I am unsure what happened to Jesse's blog, but the Wayback Machine has it here).
Furthermore, the transitions between Python code and C extensions create overhead that accumulates in complex workflows. Each time control flows from Python to a C extension, the GIL must be released and then reacquired, introducing latency. For data preprocessing pipelines or complex model architectures that frequently alternate between Python logic and optimized numerical operations, this overhead can significantly impact overall performance.
Memory Management Inefficiencies: Beyond Thread Limitation
The GIL's impact extends beyond just limiting thread execution to creating memory management inefficiencies that are particularly problematic for machine learning workloads. Python's reference counting approach "needs to be protected... from being accidentally released from memory, which is what GIL does." While this protection is crucial, it creates a memory management model that's not optimized for the large, dynamic memory allocations common in machine learning.
These inefficiencies manifest in several ways. First, the GIL's synchronization requirements add overhead to memory operations, slowing down allocation and deallocation. Second, Python's inability to efficiently parallelize memory operations means that large-scale data manipulations—common in preprocessing—often require more time and memory than they would in a truly parallel environment. Finally, the stop-the-world moments when Python needs to acquire or release the GIL create micro-pauses that, while individually small, can accumulate to significant latency in performance-critical applications.
Quantifying the GIL's Performance Impact
The performance ceiling imposed by the GIL is not theoretical—it's measurable and significant. Benchmark studies consistently show substantial performance discrepancies between single-threaded and multi-threaded Python code for CPU-bound tasks. When comparing execution times of CPU-bound tasks in sequential and parallel scenarios, "the parallel execution using multiple threads takes longer than the sequential execution. This is due to the GIL's overhead and contention, which limit the benefits of parallelism." This counterintuitive result—where adding threads actually decreases performance—highlights the GIL's profound impact.
For machine learning specifically, this translates to longer training times, reduced experimentation capacity, and less efficient resource utilization. The impact becomes especially apparent when comparing Python's performance to languages without equivalent restrictions, such as Julia or Rust, which can achieve significantly better parallelization on multi-core systems for similar workloads.
Case Studies: The GIL in Production ML Systems
Real-world machine learning applications frequently encounter GIL-related bottlenecks. One illustrative example comes from large-scale data processing pipelines at tech companies. NumPy, a foundational package for scientific computing in Python, "doesn't offer a solution to utilize all CPU cores of a single machine well, and instead leaves that to Dask and other multiprocessing solutions. Those aren't very efficient and are also more clumsy to use." This situation forces developers to implement complex workarounds that add both code complexity and deployment overhead.
Another example involves distributed training systems. While frameworks like PyTorch and TensorFlow implement distributed training capabilities, the Python code coordinating these operations still runs under GIL constraints. This can create synchronization bottlenecks when aggregating gradients or distributing updated model parameters across workers, reducing the efficiency of distributed training.
This is Not a Python Rant
I want to be abundantly clear after all of this description and deep diving, I am not saying "Python sucks" or "never use Python". The language is an amazingly expressive one and one that almost feels natural when manipulating data, in many ways more so than any other language I have had experience with. It has an almost "pick up and soar to heights" feeling to it that allows scientists, educators, and many others who self identify as "not a programmer" to do amazing things in a short period of time. Even better, it enables people who identify as "programmers" to do even bigger and better things faster. As a language to do amazing things, it has to be said that it does sit at the top and statistically it does year over year as exemplified by the TIOBE programming community index.
This blog post, and this series in total, is just trying to present the conversation that while it may be a beloved programming language that has enabled a tremendous volume of incredible things, we - as engineers - should be willing and open to:
- Be aware and communicative of the trade offs that come along with the {language, system, framework, etc.} of choice
- Be open to a reality that while a {language, system, framework, etc.} may excel at allowing us to build, explore, and prototype concepts swiftly - that does not guarantee that it is the right tool for all use cases of those concepts.
The Road Forward: Solutions and Alternatives
Despite these challenges, the machine learning community has developed several approaches to mitigate the GIL's limitations:
- Multiprocessing: The most common workaround involves using multiple Python processes instead of threads. Unlike threading, multiprocessing provides "a different interpreter to each process to run... Each process gets its own Python interpreter and memory space which means GIL won't stop it." However, this approach increases memory overhead and complicates data sharing between processes.
- Alternative Python Implementations: Implementations like Jython and IronPython don't have a GIL, allowing true parallelism. However, they often lack compatibility with key machine learning libraries or have other performance trade offs.
- Native Extensions and Cython: Writing performance-critical code in C/C++ or using Cython allows developers to release the GIL during computationally intensive operations, though it sacrifices some of Python's simplicity and readability. Furthermore there is only so much that one can move out of Python before you are realistically just writing (and maintaining) C/C++.
- Alternative Languages: Some teams are exploring languages like Julia or Rust for performance-critical machine learning components. Julia "is a language exclusively designed to address the shortcomings of Python" including the GIL's limitations on parallel computing.
- Alternative Operating Realities: Some teams, such as YetiWare, are exploring a fundamental revolution at the lowest levels of computing by challenging the very Von Neumann architecture upon which Python (and all other languages) sit atop. This could bring about a whole new realm of computing, with benefits and potentially downsides, and is worthwhile keeping tabs on.
- GIL-free Python: The most promising long-term solution may be the ongoing work to make the GIL optional in CPython. PEP 703, which the Python Steering Council intends to approve, "proposes a way to remove the GIL from Python but manages to avoid the performance impact on non-multithreaded code that affected other no-GIL Python projects."
Navigating Python's Performance Landscape
The GIL remains a significant constraint on Python's performance for machine learning and other CPU-intensive applications. Understanding its implications is essential for developing efficient machine learning systems and making informed decisions about technology stacks. While workarounds exist, they introduce additional complexity or performance trade offs that must be carefully weighed.
As we look to the future, the potential for a GIL-free Python implementation offers hope for addressing these limitations while preserving Python's ecosystem advantages. Until then, machine learning practitioners should remain aware of the GIL's impact on their workflows and consider appropriate mitigation strategies for performance-critical applications. By understanding these architectural constraints, we can better navigate the challenges of building high-performance machine learning systems in and/or with Python - it is not an all or nothing outcome.